分页
项目目录
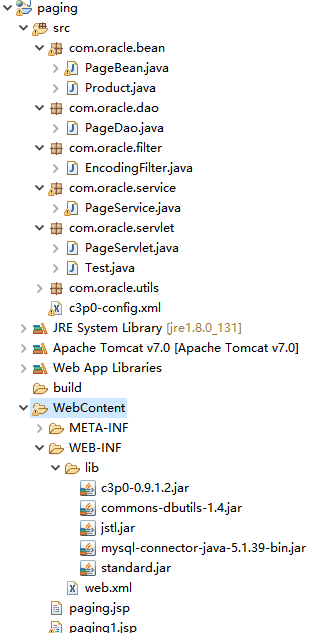
创建表
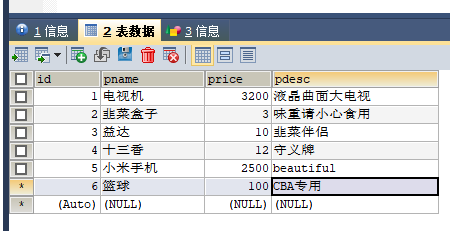
分页后台实现
- paging.jsp(发出分页查询请求)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<a href="/paging/page?pageNumber=1">分页查询</a>
</body>
</html>
- PageServlet.java(处理请求)
package com.oracle.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.oracle.bean.PageBean;
import com.oracle.service.PageService;
public class PageServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//获取前台传递的当前页
String pn = request.getParameter("pageNumber");
int pageNumber = Integer.parseInt(pn);
int pageSize = 2;
PageService ps = new PageService();
PageBean pb = ps.getPageBean(pageNumber, pageSize);
//把pb放入request域对象中
request.setAttribute("pb", pb);
//请求转发到前台页面
request.getRequestDispatcher("/paging1.jsp").forward(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
- PageService.java(编写业务逻辑)
package com.oracle.service;
import java.sql.SQLException;
import java.util.List;
import com.oracle.bean.PageBean;
import com.oracle.bean.Product;
import com.oracle.dao.PageDao;
public class PageService {
public PageBean getPageBean(int pageNumber, int pageSize) {
PageBean pb = null;
try {
// TODO Auto-generated method stub
PageDao pd = new PageDao();
//组装PageBean
pb = new PageBean(pageSize, pageNumber);
//查询当前页显示的数据
List<Product> list = pd.getProList(pb);
//查询总条数
int count = pd.getCount();
//把数据放如pagebean中
pb.setList(list);
pb.setTotalSize(count);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return pb;
}
}
- PageDao.java(操作数据库)
package com.oracle.dao;
import java.sql.SQLException;
import java.util.List;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import com.oracle.bean.PageBean;
import com.oracle.bean.Product;
import com.oracle.utils.DataSourceUtils;
public class PageDao {
public List<Product> getProList(PageBean pb) throws SQLException {
// TODO Auto-generated method stub
QueryRunner qr = new QueryRunner(DataSourceUtils.getDataSource());
String sql = "select * from product limit ?,?";
List<Product> list = qr.query(sql, new BeanListHandler<>(Product.class), pb.getIndex(), pb.getPageSize());
return list;
}
public int getCount() throws SQLException {
// TODO Auto-generated method stub
QueryRunner qr = new QueryRunner(DataSourceUtils.getDataSource());
String sql = "select coutn(*) from product";
int count = ((Long)qr.query(sql, new ScalarHandler())).intValue();
return count;
}
}
分页前台实现
- paging1.jsp(展示分页查询结果)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<table border="1px" width="60%" height="200px" align="center">
<tr>
<th>商品id</th>
<th>商品名称</th>
<th>商品价格</th>
<th>商品描述</th>
</tr>
<c:forEach items="${pb.list}" var="pro">
<tr>
<td>${pro.id}</td>
<td>${pro.pname}</td>
<td>${pro.price}</td>
<td>${pro.pdesc}</td>
</tr>
</c:forEach>
</table>
<center>
<c:forEach begin="1" end="${pb.totalCount}" step="1" var="n">
<a href="/paging/page?pageNumber=${n}">${n}</a>
</c:forEach>
</center>
</body>
</html>
- 效果
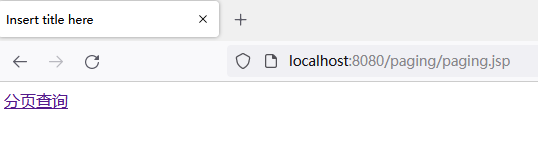
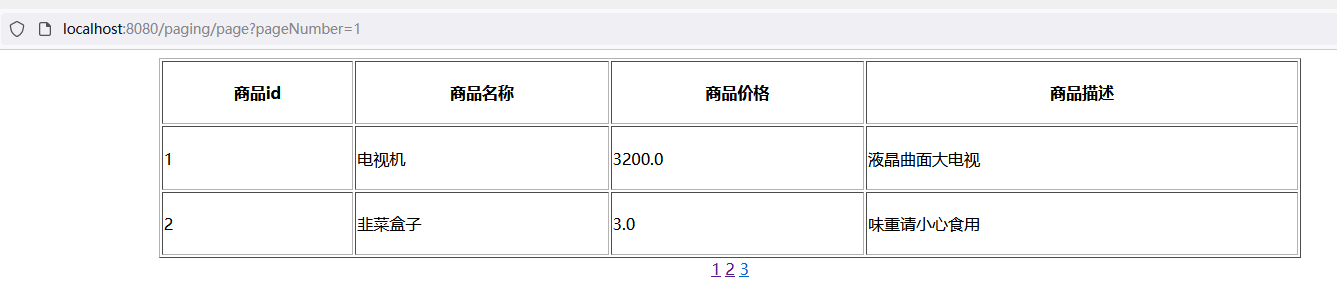